How to Automate Emails with Python for Beginners Part 2
- Jul 27, 2020
- 3 min read
What we will cover
In this blog post we will be using our knowledge of sending emails with Python to allow us to bulk send emails. This means we will form a spreadsheet with several email addresses and send a specific email message to each one.
Let's Get Started
We should begin by breaking the program down into sections allowing us to make the program more approachable.
Reading the email addresses from the spreadsheet
Sending an email to each name
Now Let's Start Coding in Python
For this program we won't have to install any additional 3rd party modules we will simply use one built-in Python module which can be imported as follows:
import csv
CSV stands for "Comma-separated values", this is essentially a file type which all spreadsheet software uses.
STEP 1 - Create a spreadsheet with Email addresses
So first of all, we will create a simple spreadsheet with only a couple Email addresses and we will start off by getting our code to read the spreadsheet and print out the email addresses on separate lines.
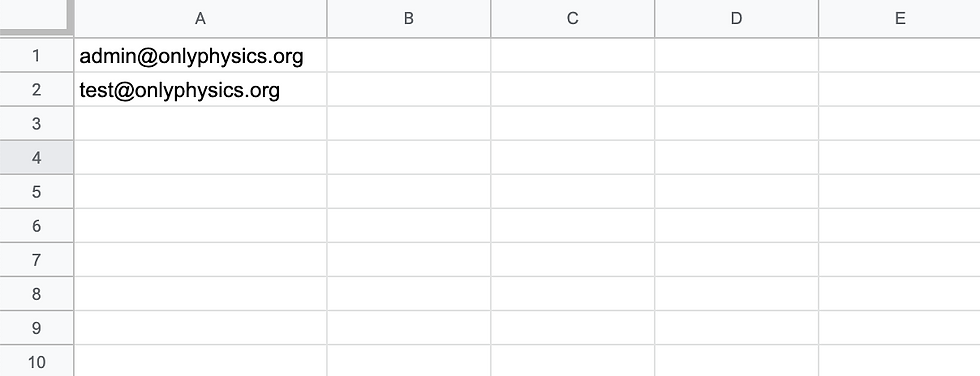
We need to save the spreadsheet as a CSV file, this can be done by giving it the '.csv' file extension. You can also do this by going to the export tab and choosing CSV file. Remember to save the spreadsheet file and the Python file at the same location!
STEP 2 - Begin the program
Then once this is completed you are ready to begin writing the first main chunk of the of the program.
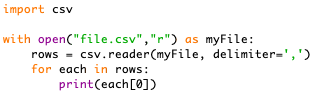
So we need to start off by reading each row from the CSV file and storing this in a Python list. A list is essentially able to store several values and in this example we are creating a list which consists of several other lists. Each list inside the main list stores the data for each row. We can simply use a for loop to print each row as its own list and then reference the first item within each row by using index numbers. In programming, 0 references the index of the first item in the list and this allows us to print the email addresses within the CSV document.
STEP 3 - Sending the Emails
Start this step by importing the modules we require when sending an email, remember to do this at the top of the file as always! So now we are able to send emails to each address by simply replacing the to_email with each address from our CSV file. In order to do this you will need to create a function with the code that was used to send emails from the previous part of this series. Here is what it should look like:
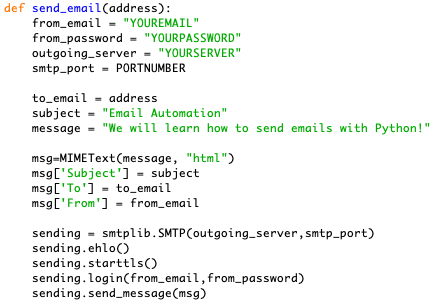
This function can be referenced at any point in the code and simply allows an email to be send to whatever address you pass in. When you replace 'print(each[0])' with 'send_email(each[0])' this will run the send_email function and stores the email address under the keyword 'address'.
Final Complete Solution!
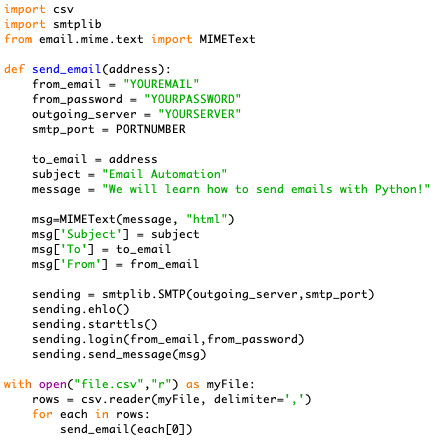
We have completed this program and it should work perfectly! If it doesn't work for you try reading the first part of this series and see if you have made any mistakes there. If not, go ahead and leave me a comment and I will get back to you straight away! Don't forget to become a member of OnlyPhysics, go sign up right now :)
Comentarios